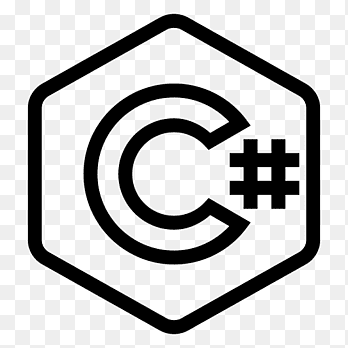
{filelink=16161}
using System;
using System.Diagnostics;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.SqlClient;
public class ListBoxEtTable : System.Windows.Forms.Form
{
SqlConnection connexion = new SqlConnection("server=(local);database=MesExemples.com;Integrated Security=SSPI");
SqlDataAdapter da = new SqlDataAdapter();
string requête= "Select ID, Société from Clients";
SqlCommand commande;
SqlDataReader dr;
System.Windows.Forms.Button btnCharger = new System.Windows.Forms.Button();
System.Windows.Forms.ListBox ListBox1 = new System.Windows.Forms.ListBox();
System.Windows.Forms.TextBox TextBox1 = new System.Windows.Forms.TextBox();
public ListBoxEtTable() {
commande = new SqlCommand(requête, connexion);
this.SuspendLayout();
this.btnCharger.Location = new System.Drawing.Point(136, 248);
this.btnCharger.Size = new System.Drawing.Size(144, 32);
this.btnCharger.Text = "Get Data";
this.btnCharger.Click += new System.EventHandler(this.Button1_Click);
this.ListBox1.Location = new System.Drawing.Point(48, 64);
this.ListBox1.Size = new System.Drawing.Size(312, 160);
this.TextBox1.Location = new System.Drawing.Point(48, 24);
this.TextBox1.Size = new System.Drawing.Size(328, 20);
this.TextBox1.Text = "Hit";
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(408, 293);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.btnCharger,
this.ListBox1,
this.TextBox1});
this.ResumeLayout(false);
}
[STAThread]
static void Main() {
Application.Run(new ListBoxEtTable());
}
private void Button1_Click(object sender, System.EventArgs e) {
connexion.Open();
commande.CommandText = requête + " where société like '" + TextBox1.Text + "%'";
dr = commande.ExecuteReader();
ListBox1.Items.Clear();
ListBox1.BeginUpdate();
while (dr.Read()){
ListBox1.Items.Add(dr.GetString(0) + " - " + dr.GetInt32(1).ToString());
}
ListBox1.EndUpdate();
dr.Close();
}
}