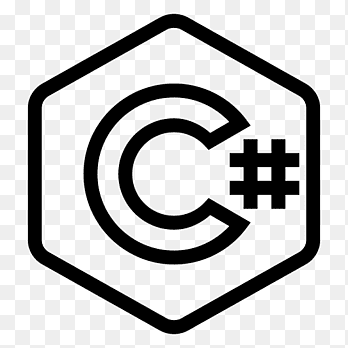
{filelink=16391}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
public class DataTableXML : Form
{
private System.Windows.Forms.Button btnRemplirLaListe;
private System.Windows.Forms.ListView MaListe;
private System.Windows.Forms.CheckBox GroupsCheckBox;
public DataTableXML()
{
InitializeComponent();
MaListe.View = View.Tile;
MaListe.TileSize = new Size(300, 50);
RemplirLaListe();
}
public static DataTable GetProducts()
{
DataSet dsStore = new DataSet();
dsStore.ReadXml("Operations.xml");
return dsStore.Tables["Clients"];
}
public static DataTable GetCategories()
{
DataSet dsStore = new DataSet();
dsStore.ReadXml("Operations.xml");
return dsStore.Tables["Clients"];
}
private void cmdRemplirLaListe_Click(object sender, EventArgs e)
{
RemplirLaListe();
}
private void RemplirLaListe()
{
MaListe.Items.Clear();
if (MaListe.Groups.Count == 0)
{
DataTable dtGroups = GetCategories();
foreach (DataRow dr in dtGroups.Rows)
{
MaListe.Groups.Add(dr["IDClient"].ToString(), dr["Entreprise"].ToString());
}
}
MaListe.ShowGroups = GroupsCheckBox.Checked;
DataTable dtProducts = GetProducts();
MaListe.BeginUpdate();
foreach (DataRow dr in dtProducts.Rows)
{
ListViewItem listItem = new ListViewItem(dr["Entreprise"].ToString());
listItem.ImageIndex = 0;
listItem.Group = MaListe.Groups[dr["IDClient"].ToString()];
listItem.SubItems.Add(dr["IDClient"].ToString());
MaListe.Items.Add(listItem);
}
if (MaListe.Columns.Count == 0)
{
MaListe.Columns.Add("Entreprise", 100, HorizontalAlignment.Left);
MaListe.Columns.Add("IDClient", 100, HorizontalAlignment.Left);
}
MaListe.EndUpdate();
}
private void NewView(object sender, System.EventArgs e)
{
MaListe.View = (View)(((Control)sender).Tag);
}
private void listView_ColumnClick(object sender, ColumnClickEventArgs e)
{
ListViewItemComparer sorter = MaListe.ListViewItemSorter as ListViewItemComparer;
if (sorter == null)
{
sorter = new ListViewItemComparer(e.Column);
MaListe.ListViewItemSorter = sorter;
}
else
{
if (sorter.Column == e.Column && !sorter.Descending)
{
sorter.Descending = true;
MaListe.Sorting = SortOrder.Descending;
}
else
{
MaListe.Sorting = SortOrder.Ascending;
sorter.Descending = false;
sorter.Column = e.Column;
}
}
MaListe.Sort();
}
private void cmdResizeColumns_Click(object sender, EventArgs e)
{
MaListe.AutoResizeColumns(ColumnHeaderAutoResizeStyle.HeaderSize);
}
private void listView_SelectedIndexChanged(object sender, EventArgs e)
{
if (MaListe.SelectedItems.Count > 0)
MessageBox.Show(MaListe.SelectedItems[0].SubItems[1].Text);
}
private void chkGroups_CheckedChanged(object sender, EventArgs e)
{
RemplirLaListe();
}
private void InitializeComponent()
{
this.btnRemplirLaListe = new System.Windows.Forms.Button();
this.MaListe = new System.Windows.Forms.ListView();
this.GroupsCheckBox = new System.Windows.Forms.CheckBox();
this.SuspendLayout();
//
// btnRemplirLaListe
//
this.btnRemplirLaListe.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
this.btnRemplirLaListe.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.btnRemplirLaListe.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.btnRemplirLaListe.Location = new System.Drawing.Point(68, 345);
this.btnRemplirLaListe.Name = "btnRemplirLaListe";
this.btnRemplirLaListe.Size = new System.Drawing.Size(114, 24);
this.btnRemplirLaListe.TabIndex = 7;
this.btnRemplirLaListe.Text = "Remplir La Liste";
this.btnRemplirLaListe.Click += new System.EventHandler(this.cmdRemplirLaListe_Click);
//
// MaListe
//
this.MaListe.AllowColumnReorder = true;
this.MaListe.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.MaListe.FullRowSelect = true;
this.MaListe.GridLines = true;
this.MaListe.Location = new System.Drawing.Point(7, 8);
this.MaListe.MultiSelect = false;
this.MaListe.Name = "MaListe";
this.MaListe.Size = new System.Drawing.Size(259, 331);
this.MaListe.Sorting = System.Windows.Forms.SortOrder.Ascending;
this.MaListe.TabIndex = 6;
this.MaListe.UseCompatibleStateImageBehavior = false;
this.MaListe.SelectedIndexChanged += new System.EventHandler(this.listView_SelectedIndexChanged);
this.MaListe.ColumnClick += new System.Windows.Forms.ColumnClickEventHandler(this.listView_ColumnClick);
//
// GroupsCheckBox
//
this.GroupsCheckBox.Location = new System.Drawing.Point(0, 0);
this.GroupsCheckBox.Name = "GroupsCheckBox";
this.GroupsCheckBox.Size = new System.Drawing.Size(104, 24);
this.GroupsCheckBox.TabIndex = 0;
//
// DataTableXML
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(267, 381);
this.Controls.Add(this.MaListe);
this.Controls.Add(this.btnRemplirLaListe);
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.Name = "DataTableXML";
this.Text = "Exemple ListView Avec XML";
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new DataTableXML());
}
}
public class ListViewItemComparer : System.Collections.IComparer
{
private int column;
public int Column
{
get { return column; }
set { column = value; }
}
private bool numeric = false;
public bool Numeric
{
get { return numeric; }
set { numeric = value; }
}
private bool descending = false;
public bool Descending
{
get { return descending; }
set { descending = value; }
}
public ListViewItemComparer(int columnIndex)
{
Column = columnIndex;
}
public int Compare(object x, object y)
{
ListViewItem listX, listY;
if (descending)
{
listY = (ListViewItem)x;
listX = (ListViewItem)y;
}
else
{
listX = (ListViewItem)x;
listY = (ListViewItem)y;
}
if (Numeric)
{
decimal valX, valY;
Decimal.TryParse(listX.SubItems[Column].Text, out valX);
Decimal.TryParse(listY.SubItems[Column].Text, out valY);
return Decimal.Compare(valX, valY);
}
else
{
return String.Compare(
listX.SubItems[Column].Text, listY.SubItems[Column].Text);
}
}
}
//Fichier:Operation.xml
/*
CCLiege
Genital
Frederic Moulard
D.G Commercial
15 Rue X
Paris
2025
France
20-30-14-30-65
20-30-14-30-66
CCVerge
XMey
Herve Banste
PDG
30, rue des Galliers
Marseille
13008
France
32-15-22-16-63
32-15-22-16-64
CCDerge
Fourgon
Samuel Etienne
D.G Commercial
Cener Str. 213
Berlin
12209
Allemagne
044-2014587
044-2014588
*/